Caching fetch requests within your edge function can reduce the load on your origins and deliver content faster to your users. It may also mitigate timeout issues due to an edge function exceeding the walltime limit.
In this section, we’ll cover how to use the caching properties as part of the
fetch()
method. These properties are specified per fetch request and are completely separate from the caching properties specified in routes.[js|ts]
. This guide also covers the default caching behavior of fetch requests and how origin cache directives affect caching.Order of Operations
When a fetch request is made, the CDN will first check for a cached response. If found, it will be served from the cache. Otherwise, the request will be sent to the origin. Edgio will cache the response when any of the following conditions are met:
- Custom caching properties are specified in the
edgio
object. - A valid
Cache-Control
header is present in the origin response. - The origin response satisfies the criteria for the default cache behavior.

If the fetch request is instructed to bypass the cache, the request will be sent directly to the origin and the response will not be cached.
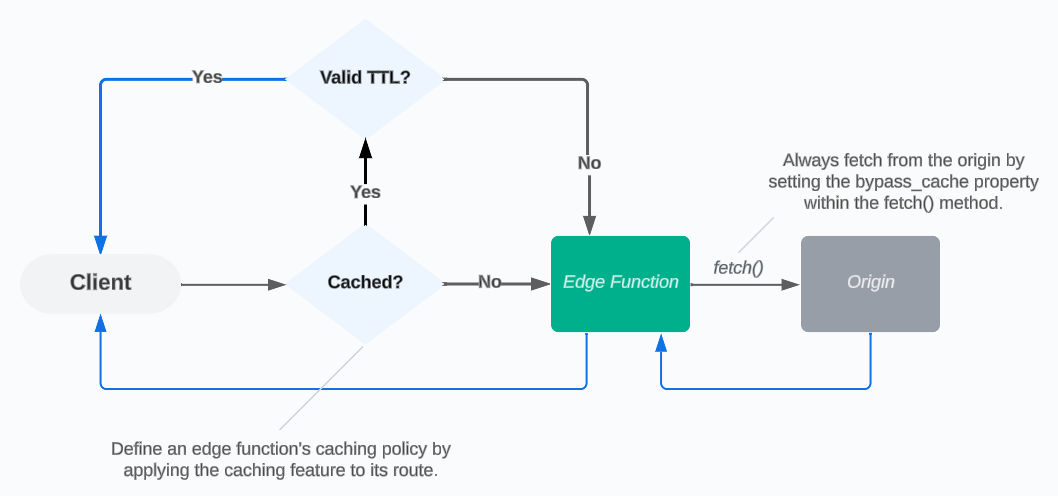
Caching Scenarios
Default 5 Minute Caching
JavaScriptedge-functions/main.js
1export async function handleHttpRequest(request) {2 // - assume no `cache-control` directives from the origin3 // - response is stored in cache for 5 minutes4 // - subsequent fetch requests to this same path will be served from cache for 5 minutes5 const response = await fetch('https://example.com/foo/bar', {6 edgio: {7 origin: 'web'8 }9 });1011 return response;12}
Origin Cache-Control Header
JavaScriptedge-functions/main.js
1export async function handleHttpRequest(request) {2 // - assume `cache-control` directives from the origin are valid and respected3 // - response is stored in cache based on the origin `cache-control` directives4 // - subsequent fetch requests to this same path will be served from cache for the defined TTL5 const response = await fetch('https://example.com/foo/bar', {6 edgio: {7 origin: 'web'8 }9 });1011 return response;12}
Custom Caching Properties
JavaScriptedge-functions/main.js
1export async function handleHttpRequest(request) {2 // - origin `cache-control` directives are ignored3 // - response is stored in cache for 10 minutes4 // - subsequent fetch requests to this same path will be served from cache for 10 minutes5 const response = await fetch('https://example.com/foo/bar', {6 edgio: {7 origin: 'web',8 caching: {9 max_age: '10m'10 }11 }12 });1314 return response;15}
Caching fetch() Requests
The following sample code shows how to define a custom caching policy for a fetch request. The caching properties are specified in the
edgio
object, which is passed as the second argument to the fetch()
method.JavaScript
1const resp = await fetch('https://your-server.com/some-path', {2 edgio: {3 origin: 'web',4 caching: {5 max_age: '1d',6 stale_while_revalidate: '1h',7 tags: 'apple banana',8 bypass_cache: false,9 },10 },11});
Caching Properties
max_age
: Specifies the maximum amount of time that a fetched response is considered fresh. This value is set as a duration string, which is a number followed by a time unit character. Supported time unit characters ared
for days,h
for hours,m
for minutes, ands
for seconds. For example,"1h"
represents 1 hour. This setting overrides themax-age
directive in theCache-Control
header of the origin response if present.stale_while_revalidate
: Specifies the amount of time a stale response is served while a revalidation request is made in the background. This value is also set as a duration string similar tomax_age
. This setting overrides thestale-while-revalidate
directive in theCache-Control
header of the origin response if present.tags
: Allows you to specify a space-separated list of tags for the cached object, which can later be used for cache purging as surrogate keys. Each tag should be a string without spaces.bypass_cache
: A boolean value that, when set totrue
, bypasses the cache for the fetch request, ensuring the request is sent directly to the origin and the response is not stored in the cache.
These caching properties provide you with granular control over how your fetch requests are cached and served, allowing you to optimize the performance of your edge function.
Cache Behavior of fetch() Requests
Edge function fetch requests are cached at the edge for 5 minutes under the following conditions:
- The response from the origin does not include a
Cache-Control
header. - The response is deemed cacheable based on our default caching policy.
This means that if you make a fetch request to the same URL within 5 minutes, the response will be served from the cache instead of going to the origin. This behavior can be overridden by specifying the
bypass_cache
option described above. Cache directives from the origin response will also be respected as follows:- If the origin responds with a
Cache-Control
HTTP header containing valid directives, these directives will be respected. For example:- With
Cache-Control: max-age=60, s-maxage=900
, the fetch request will be cached for 15 minutes, considerings-maxage=900
. - With
Cache-Control: max-age=600
, the response will be cached for 10 minutes, consideringmax-age=600
. - With
Cache-Control: no-store, no-cache
, the response will not be cached.
- With
- If the
Cache-Control
header is not present for a cache-eligible response, the CDN will check for theExpires
header. - If the response is cached based on the above logic, subsequent fetch requests will be served from cache until the cached response has expired or been purged. At which point, the fetch request will go to the origin.