This page provides an overview of methods available to upload and share your Origin Storage content.
Upload Content
There are multiple ways to ingest content using Origin Storage. Choose from the options below:
- Origin Storage APIs: Use Origin Storage directly from your application.
- Control Portal: This is the easiest and most expeditious way to get started. If you don’t like using a command line, this is for you.
- Java FTP and API client
- Rsync over SSH. You can use this method directly from the command line.
Whenever possible it is recommended that customers use the Origin Storage Application Programming Interface (API) due to its feature-rich ingest workflow and its superior performance.
Uploading Using the Origin Storage API
This section provides an end-to-end example application to jump-start client-side integration with Origin Storage. This section shows how to create a token, upload a file over HTTP, and verify that the file was correctly uploaded.
This HTTP Example ties information together, providing a complete working sample.
Step 1: Establish a Token
You can use HTTP or JSON-RPC.
HTTP Example
Use the Python requests library.
HTTP
1>>> import requests23>>> auth_headers = { 'X-Agile-Username': 'guest', 'X-Agile-Password': 'password' }45>>> url = 'http://{Account Name}.upload.llnw.net/account/login'67>>> r = requests.post(url, headers=auth_headers, verify=False)89>>> token = r.headers['x-agile-token']1011>>> print token12137c164371-d581-4068-8ce1-4d17b6a9d8a3
JSON-RPC Example
Use the Python jsonrpclib library.
JSON
1>>> import jsonrpclib23>>> api = jsonrpclib.Server('http://{Account Name}.upload.llnw.net/jsonrpc2')45>>> token,uid_gid = api.login('guest', 'password')67>>> print token89f3037573-2a6f-4042-ab8f-82d6823b0480
Step 2: Upload a File Over HTTP
Always use HTTP for file uploads! This example uses /post/raw submitted with the Python requests library.
HTTP
1>>> import requests23>>> file_to_upload = "test.mp3"45>>> upload_headers = { 'X-Agile-Authorization': token, 'X-Agile-Basename': file_to_upload, 'X-Agile-Directory': '/' }67>>> with open(file_to_upload, 'rb') as filesrc:89 r = requests.post( 'http://{Account Name}.upload.llnw.net/post/raw', data=filesrc, headers=upload_headers)
Step 3: Verify the Upload was Successful
You can use HTTP or JSON-RPC.
HTTP Example
Look at the headers returned from the request. The /post/raw request returns the upload status in the X-Agile-Status header. A value of 0 means success.
HTTP
1>>> print r.headers23{'x-agile-status': '0', 'content-length': '20', 'access-control-allow-methods': 'OPTIONS',45'content-type': 'text/xml;charset=utf-8', 'content-encoding': 'gzip',67'x-agile-checksum': 'ce2a9d3bdec04c3577164d067e479958b50e246f17aaa098d0fad9f150eb4465',89'vary': 'Accept-Encoding', 'server': 'Apache', 'date': 'Tue, 03 Mar 2015 19:31:18 GMT',1011'x-agile-path': '/{Account Name}/test.mp3', 'access-control-allow-origin': '*',1213'access-control-allow-headers': 'X-Agile-Authorization, X-Content-Type', 'x-agile-size': '2055946'}
Or, you can issue a HEAD request against the object and look at the headers returned and the overall response code (200=OK). Here is an example using the Python requests library:
HTTP
1>>> r = request.head('http://{Account Name}.upload.llnw.net/test.mp3')23>>> print r45<Response [200]>67>>> print r.headers89{‘content-length”, ‘2055946’, ‘x-agile-checksum’: 'ce2a9d3bdec04c3577164d067e479958b50e246f17aaa098d0fad9f150eb4465',1011’last-modified’: ‘Thu, 12 Apr 2012 19:31:18 GMT’, ‘connection’: ‘keep-alive’, ‘date’:’Tue, 03 Mar 2015 19:31:18 GMT’,1213 ‘accept-ranges’: ‘bytes’, ‘server’:’nginx/1.0.12, ‘content-type’: ‘audio/mpeg’}
JSON-RPC Example
Use the stat function to get information on the uploaded file. The stat function has an optional ‘detail’ parameter. Pass True for extended information, or pass False (or omit) for an abbreviated response. A value of 0 in the returned code field indicate success. Other values indicate failure. You can also look at other fields such as size and compare them to known values.
JSON
1>>> api.stat(token, '/test.mp3')23{u'mtime': 1425411089, u'code': 0, u'type': 2, u'ctime': 1425411089, u'size': 2055946}45>>> api.stat(token, '/test.mp3',True)67{u'mimetype': u'audio/mpeg', u'code': 0, u'uid': 1000009,89u'checksum': u'ce2a9d3bdec04c3577164d067e479958b50e246f17aaa098d0fad9f150eb4465',1011u'gid': 1000003, u'mtime': 1425411089, u'size': 2055946, u'type': 2, u'ctime': 1425411089}
Complete HTTP Example
This section ties information together, providing a complete Python working sample that you can quickly and easily copy and paste into a Python file.
Python
1import requests23myusername = "guest"45mypassword = "password"67file_to_upload = "test.mp3"8910auth_headers = { 'X-Agile-Username': myusername, 'X-Agile-Password': mypassword }1112url = 'http://{Account Name}.upload.llnw.net/account/login'1314r = requests.post(url, headers=auth_headers, verify=False)1516token = r.headers['x-agile-token']171819upload_headers = { 'X-Agile-Authorization': token, 'X-Agile-Basename': file_to_upload, 'X-Agile-Directory': '/' }2021with open(file_to_upload, 'rb') as filesrc:2223 r = requests.post('http://{Account Name}.upload.llnw.net/post/raw', data=filesrc, headers=upload_headers)242526print r.headers
Uploading Using the Control Portal
You can upload using the drag-and-drop capability in the Control Portal.
Step 1: Log Into Control
- Go to https://control-new.edg.io/acontrol/#/login.
- Log in using the credentials and shortname/account information you received in your Edgio Welcome Letter.
Step 2: Create Directories
If needed, you can create target directories using the Origin Storage API or the Control portal.
Origin Storage API
API | Additional Information in the Origin Storage API Reference Guide |
---|---|
makeDir or makeDir2 | Working with Directories in the JSON-RPC Interface |
/post/directory | Working with Directories in the HTTP Interface |
Control Portal
See Creating Folders in the Origin Storage Console User Guide.
Step 3: Upload Content
To upload content using the Control Portal, please see Uploading Files in the Control Portal User Guide.
If you would like to upload content using another method (API, FTP, etc.), see the links to the guides in Choose an Ingest Method.
Uploading Using Rsync over SSH
Rsync is a software application for Unix-like and Windows systems which synchronizes files and directories from one location to another, while minimizing data transfer using delta encoding, when appropriate. An important feature of Rsync not found in most protocols is that mirroring takes place with only one transmission in each direction. Rsync can copy or display directory contents and copy files, optionally using compression and recursion.
- Rsync over SSH is available only with pre-configured Source IP Access Control List (ACL) provisioning
- Rsync over SSH supports both pre-configured SSH authorized keys and basic password authentication
- Rsync options that trigger mkstemp() system calls are not supported
- The file system path accessing Origin Storage via SCP is /content
- must be specified as the prefix when using Rsync to ingest files (e.g.,
Rsync filename llp:/content/path
)
- must be specified as the prefix when using Rsync to ingest files (e.g.,
Rsync Options
The following options are known to work. In some cases, the options are ignored because asset ownership and permissions are dictated by the Origin Storage configuration.
The
-W
, and –inplace
options are required.Usage | Abbreviated Option | Option | Description |
---|---|---|---|
required | -W | -whole-file | copy files whole (without Rsync algorithm) |
required | -inplace | update destination files in-place (see man page) | |
optional | -q | -quiet | suppress non-error messages |
optional | -no-motd | suppress daemon-mode MOTD (see man page caveat) | |
optional | -r | -recursive | recurse into directories |
optional | -u | -update | recurse into directories |
optional | -O | -omit-dir-times | omit directories when preserving times |
optional | -i | -itemize-changes | output a change-summary for all updates |
optional | -r | -recursive | recurse into directories |
optional | -t | -times | preserve times |
optional | -u | -update | skip files that are newer on the receiver |
required | -v | -verbose | increase verbosity |
optional | -size-only | skip files that match in size | |
optional | -progress | show progress during transfer | |
optional | -stats | give some file-transfer stats | |
ignored | -g | -group | preserve group |
ignored | -o | -owner | preserve owner (super-user only) |
ignored | -p | -perms | preserve permissions |
Upload Example
This example shows how to sync files from a local machine to Origin Storage.
Directory Structure
Here is a simple directory structure:

Rsync Command
Here is the command to sync the directories using Rsync over SSH:
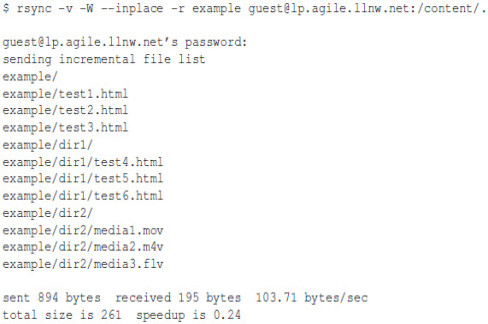
Retrieve and Share Your Content
After you upload content, you have several options for retrieving and sharing content:
- Use the Control Portal:
- To retrieve content, please see Downloading Files in the in the in the Control Portal User Guide.
- To share content via email, please see Getting Direct Links to Files in the in the in the Control Portal User Guide.
- To download and share the content via a CDN, consult your CDN provider. Origin Storage can act as origin storage for any CDN. If Edgio Content Delivery is your CDN of choice, please see Configuring Caching and Delivery in the Control Portal User Guide.
Do not attempt to directly download content from Origin Storage using FTP, SFTP, FTPS, SCP, or rsync because doing so can negatively impact other system processes. To download content, use an HTTP GET request.